Full disclosure: I am a Ruby and Java developer.
Everyone has their favorite pet languages (myself included) and I realize that this is a topic that evokes some feelings among my peers. In recent years, I strove to be more agnostic about tools and languages. While I try to be careful about making predictions, the languages and tools that are relevant now may fall out of favor in the industry in a few years. I’ll try stick to what I discovered, but I realize that my perception is colored by my experience.
I reviewed several indexes and articles including TIOBE, Transparent Language Popularity Index, IEEE Spectrum: Top Programming Languages, PopularitY of Programming Language Index, RedMonk Programming Language Rankings, Programming Language Popularity Chart and GitHub Language Trends and Fragmenting Landscape
While there was correlation between highly ranked languages between the indexes, none of them were the same. Several metrics were used including number of open source projects, number of lines of code, number of tracked bugs / issues, number of tutorials and numbers of search engine searches. The list goes on and on. How these metrics were weighted had a big impact on the overall ranking results.
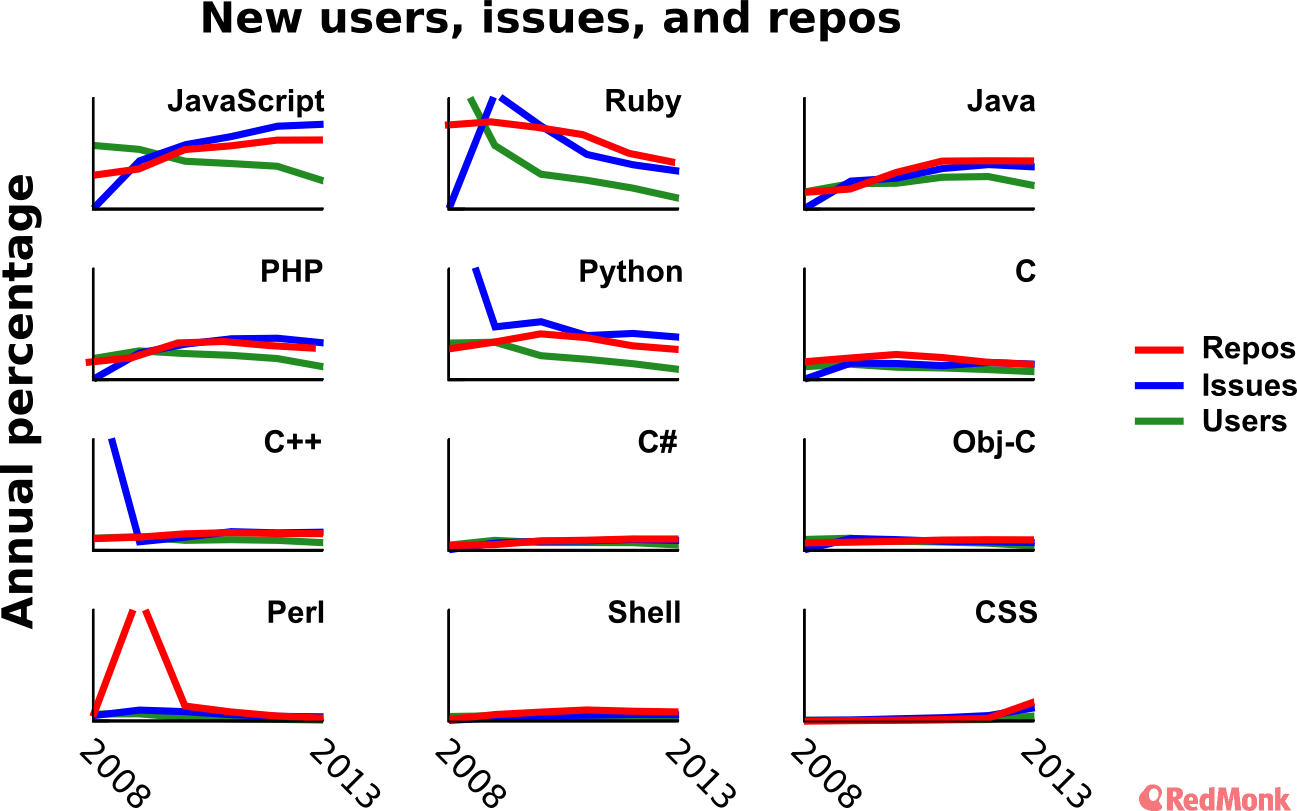
In alphabetical order some of the most highly ranked languages are C, C#, C++, Java, JavaScript, Objective-C, PHP, Perl, Python, Ruby and Visual Basic. About half the indexes I reviewed had C and Java in the top two spots.